Top 5 Programming language need to learn in 2020
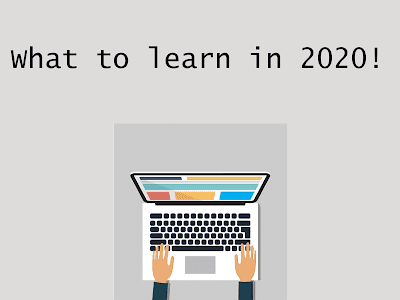
Top 5 Programming Languages need to learn in 2020 If you're new to software development. The first question that comes to mind is where to start? This is probably true! There are hundreds of choices. How are you going to discover that this is the language I want to learn? What will be the most appropriate for you, your interests and your professional goals? One of the easiest ways to choose the best programming language to learn in 2020 is to listen to what the market says. Where the technology trend is heading ... What to learn in 2020 1. JavaScript Without Javascript, you cannot think of interactive web pages. That is the main reason JavaScript is so much popular among software developers. Today, it seems impossible to be a software developer without the use of JavaScript. First from the list is JavaScript, it seems impossible to imagine software development without JavaScript. In Stack Overflow 2018 for developers, JavaScript is the most widely used by developers in t...